Merchant API
The Bitcoin SV network has introduced a setup of specifications that allow businesses and individuals to have direct interactions with miners. The motivation for this has to do with how the Bitcoin P2P network of nodes propagate transactions, and how those transactions eventually get handed off to a miner.
The Merchant API should be seen as an optimization for application developers who need a higher level of predictability w.r.t transaction fees and transaction propagation. The Merchant API exposes two primary interfaces:
- Fee Discovery - This allows the customer to have real-time transaction-fee pricing data, directly from a miner.
- Direct Transaction Submission - Allows a customer to submit their transactions directly to their chosen miner, bypassing the outer edges of the Bitcoin P2P network.
Architecture
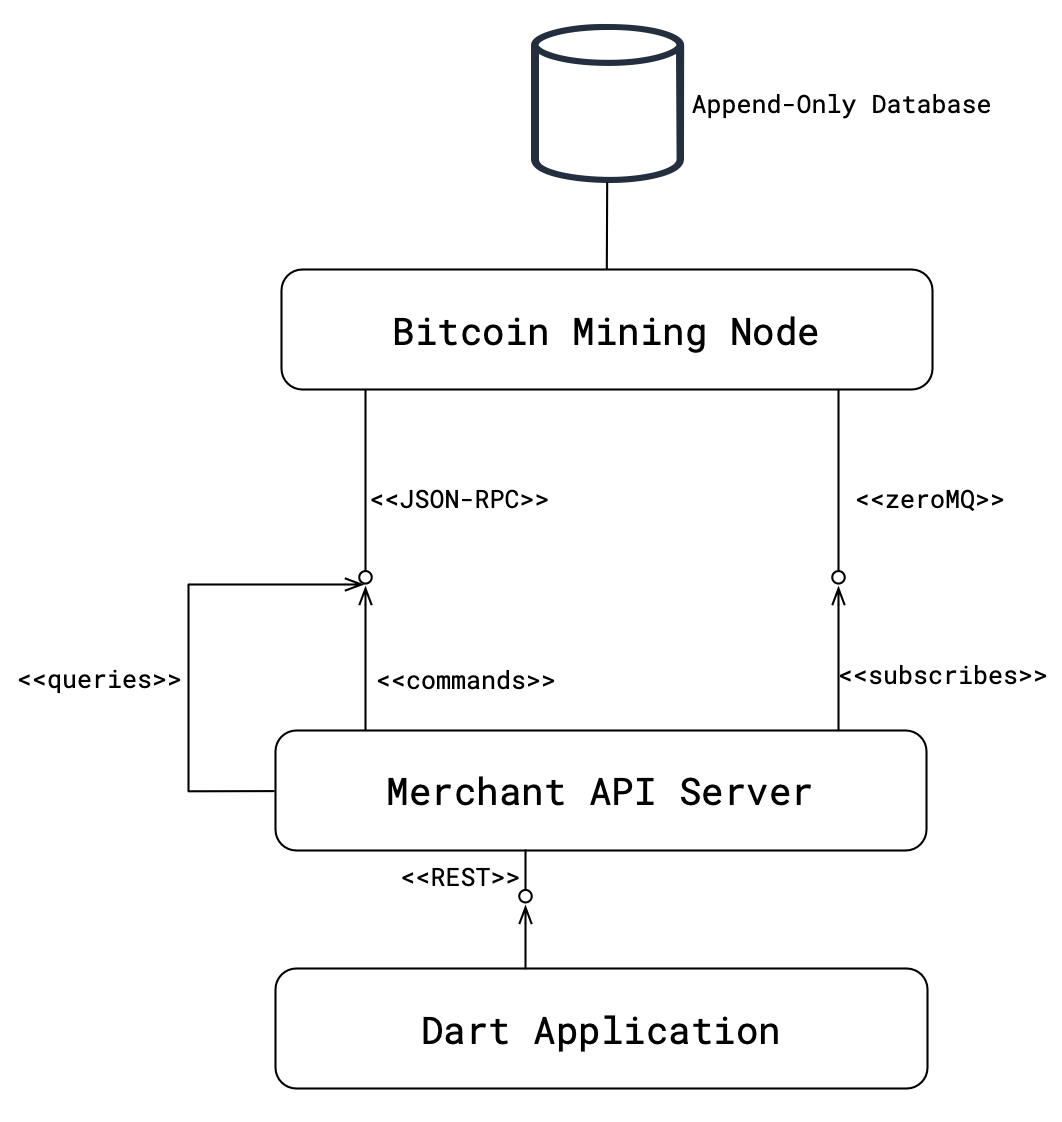
Figure 1: Connecting to the Merchant API
You would have noticed that the Merchant API now disintermediates our communication with the larger Bitcoin Network. The Merchant API Server is typically co-located with the miner’s own infrastructure. This is because miners are the entities responsible for providing the actual “Transaction Processing” or “Timestamping” service for the network. This exposes a lot of economic benefit for the application developer; for one, miners a.k.a “Transaction Processors” can now compete for the business of specific application developers. E.g. offering specific competitive fee-rates for bulk-transaction processing.
The API Specification
The Merchant API is available as a BRFC (bitcoin-request-for-comments) document. As and RFC, it lays out a specific API that miners need to implement to gain RFC compatibility. Being a BRFC also means that once the developer implements the client-side code for the API,that code becomes portable across Bitcoin SV node operators that support the Merchant API specification. Read more about the Merchant API BRFC.
A reference implementation of the Merchant API is available on Github. This is primarily targeted at miners.
Connect
To connect to the Merchant API, we will need an endpoint. At the time of writing The following node operators support the Merchant API specification.
Node Operator | URL |
---|---|
MatterPool | https://developers.matterpool.io/#merchant-api |
DotWallet | https://developers.dotwallet.com/en/docs/push/merchant |
Taal | https://www.taal.com/taal-orchestrator/mapi/ |
WhatsOnChain (API aggregator) | https://developers.whatsonchain.com/#merchant-api-beta |
No API keys are required to access the above services. The APIs require regular HTTP GET and POST requests. These should be familiar semantics to the application developer. If you are beginning to get excited, wait until we hit the section on Generic API Providers.
The Merchant API uses two important specifications as part of the protocol :
It is important to read and understand the above two specifications. The following code sample(s) will assume familiarity with the JSON Envelope and Fee Spec BRFCs.
Interact
For the purposes of our code samples, we will be connecting via the MatterPool Merchant API.
Obtaining a fee quote
void getFeeQuote() async {
var url = 'https://merchantapi.matterpool.io/mapi/feeQuote';
var client = http.Client();
var response = await client.get(url, headers: {
HttpHeaders.contentTypeHeader : ContentType.json.mimeType
});
var jsonResponse = response.body.toString();
var resultMap = jsonDecode(jsonResponse) as Map<String, dynamic>;
var jsonPayload = jsonDecode(resultMap['payload']);
var feeData = jsonPayload['fees'];
print(feeData);
}
Broadcast a transaction
Unfortunately there does not currently exist any TestNet implementations of the Merchant API spec. I will therefore leave the implementation of a HTTP POST request to submit a transaction as an exercise for the reader.